Disallow global package installs, use venv and embrace pipx
I never install packages i want to use globally, it always leads to problems due to pollution of my global environment. However i do use pipx
to do this, more about this later.
I will showcase commands that are agnostic to all platforms, they might not always work for you depending on your setup. Notably windows uses
py -m pip
and some Linux installs usespip3
. I will just usepip
here, replace the command to fit your setup.py
is the python launcher and is installed by default on windows.
pip3
is a command often used on Linux and macOS, it is a shorthand forpython3 -m pip
, for many years python2 and python3 was part of all Linux installations, you historically differentiated them with the version number. Now you can probably just usepython
Disabling global pip installs
pip config set global.require-virtualenv True
~ on ☁️ eivind.teig@amedia.no
❯ pip config set global.require-virtualenv True
Writing to /home/eivl/.config/pip/pip.conf
That created a /home/eivl/.config/pip/pip.conf
file with this content:
[global]
require-virtualenv = True
Now if i try to run pip install
outside of a virtual environment, i will see this:
❯ pip install httpx
ERROR: Could not find an activated virtualenv (required).
This is wonderful! Now I do not have to worry about accidentally pip installing packages globally.
You can of course set an environment variable in your shell config file (.bashrc, .zshrc, etc):
PIP_REQUIRE_VIRTUALENV=true
But I need global packages installed!
Before I share the solution for this, know that you are temporarily able to install packages:
PIP_REQUIRE_VIRTUALENV=false pip install --upgrade pip
I have this as a shell function, change it how you see fit.
PIP_REQUIRE_VIRTUALENV=false python -m pip install \
--disable-pip-version-check \
--no-compile \
--upgrade \
pip \
setuptools \
virtualenv \
virtualenvwrapper \
wheel \
There is frustration on all sides here, I know and I wish that the one good way to solve this was a tool that shipped with python itself. There are so many tools i want to use in the command line, and with my global pip install disabled, how can i access those without the need to activate a virtual environment?
Here is where pipx enters the fray and this resolves our problem. I now use pipx for all my global installs and I hardly ever have to force global package installations anymore.
Use the link and documentation to see the recommended way to install pipx
.
Using pipx
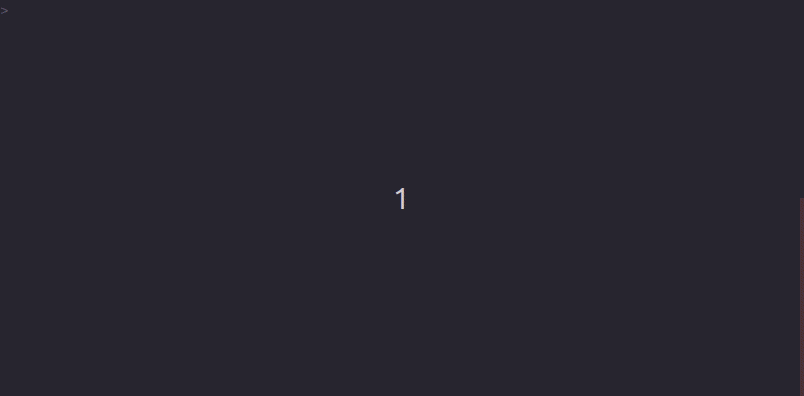
Created with Terminalizer, let me know if you wish to see a demo of this excellent tool.
pipx creates a virtual environment and installs packages used in the CLI there.
ls .local/pipx/venvs/cowsay
bin include lib lib64 pipx_metadata.json pyvenv.cfg
ls
shows the content of a folder, and the bin folder contains a script called cowsay
(like the package we installed above) and it will call the python app from within the virtual environment. It looks like this:
Here is the content of the file pipx creates, it activates the virtual environment and run the main entry-point of the package.
#!/home/eivl/.local/pipx/venvs/cowsay/bin/python
# -*- coding: utf-8 -*-
import re
import sys
from cowsay.__main__ import cli
if __name__ == '__main__':
sys.argv[0] = re.sub(r'(-script\.pyw|\.exe)?$', '', sys.argv[0])
sys.exit(cli())
This is how this issue is solved, and I would like to know if you have any feedback or otherwise details to share.